API Documentation
The API endpoint allows users to retrieve images of logos for specific merchants or store domains.
Flowchart
The below diagram shows how the process should work:
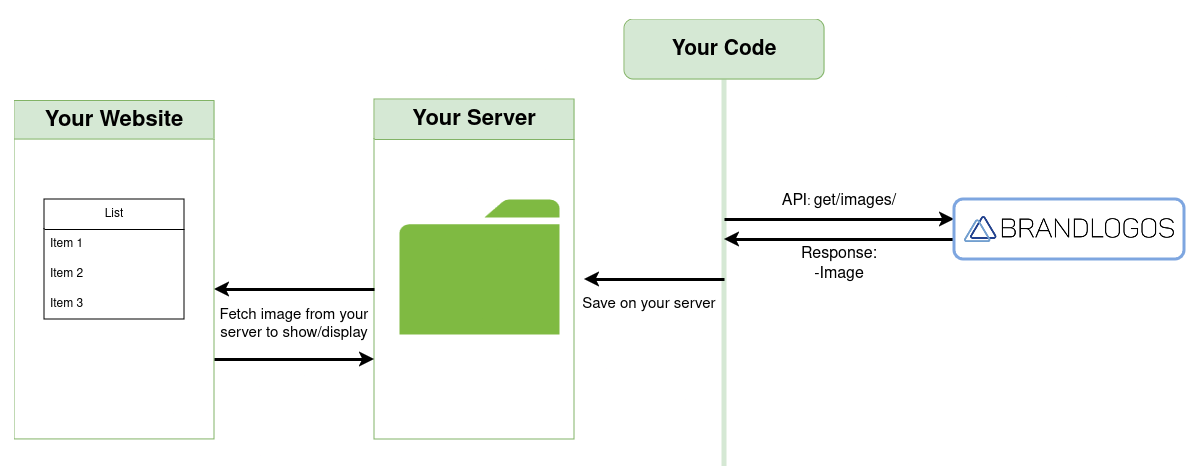
Explanation
To initiate the process, you need to develop a script or code that calls api/get/images/.
Upon receiving the request, the BrandLogos API will provide the desired image, which should then be saved on your server. Subsequently, your website can access the server to fetch and display the image as required.
API Endpoint
GET
https://brandlogos.org/api/get/images/
Sample Request:
https://brandlogos.org/api/get/images/?brand=[brand_name]&size=[size]&format=[format]&token=[token]&domain=[domain]
Request Parameters:
- brand (required) (string)
- This parameter represents the brand’s domain for which you want to fetch the logo. Example: amazon.com
- token (required) (string)
- Generate a token using the SHA256 hash of your BrandLogos API key and the brand name. Example (For PHP): hash('sha256', 'your_brandlogos_key'. 'brand_name')
- domain (required) (string)
- Enter the exact domain of your registered website. This should match the domain you have entered in your BrandLogos account. Example: yourwebsite.com
- size (optional) (string)
- Possible Values: horizontal, square
- Default Value: square
- format (optional) (string)
- Possible Values: svg, png, webp
- Default Value: svg
Success Response:
- result: You will receive an image of the brand’s logo.
Error Response:
- result: false
- error_message: (string) Reason for failure
Sample Code
PHP Script to Get Brand Image
<?php
// ========== CONFIG ==========
$API_KEY = '[YOUR_API_KEY]'; // Enter your API Key
$size = 'horizontal'; // You can use one out of two available sizes. (horizontal, square)
$format = 'png'; // You can use one out of three formats. (svg, png, webp)
$domain = '[YOUR_WEBSITE]'; // Your Website. With which you have registered on BrandLogos.Org
// You have to derive this as per your requirement. (Basically it should be the brands for which you don't have logos already.)
$brands = array("amazon.com","flipkart.com","firstcry.com");
// ========== LOOP ON BRANDS TO FETCH LOGO ONE BY ONE ==========
foreach($brands as $brand){
// Create token
$token = hash('sha256', $API_KEY . $brand);
// ========== CALL THE API TO GET IMAGE ==========
$url = "https://brandlogos.org/api/get/images/?brand=" . $brand . "&size=" . $size . "&format=" . $format . "&token=" . $token . "&domain=" . $domain;
// Fetch Response here...
$response = file_get_contents($url);
// Check if we get Error Or Image in response
if(str_contains($response, "result")){
$response = json_decode($response, true);
// Handle Error Here...
echo "<br>We encountered an error!!" . $response['error_message'];
} else{
// Save Image On Server...
file_put_contents("/path/to/folder/" . $brand . ".png", $response);
}
}
?>